반응형
문제
출처: https://www.acmicpc.net/problem/14499
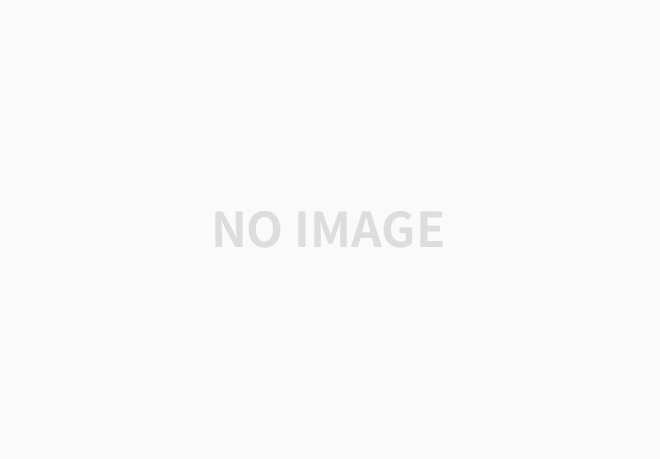
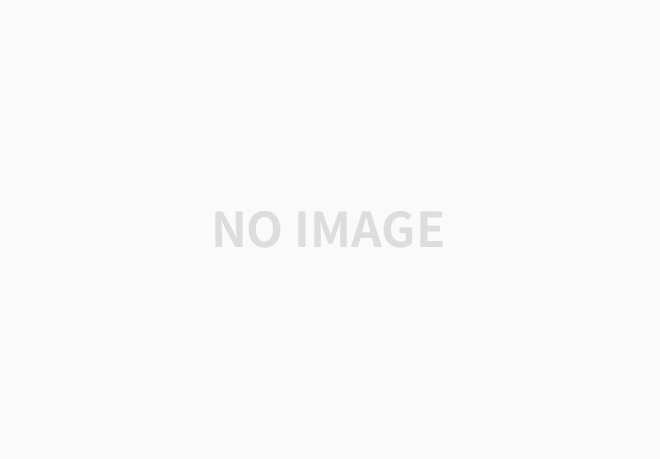
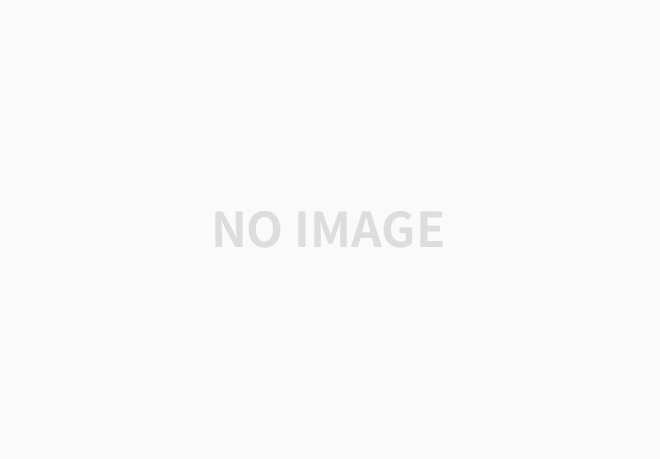
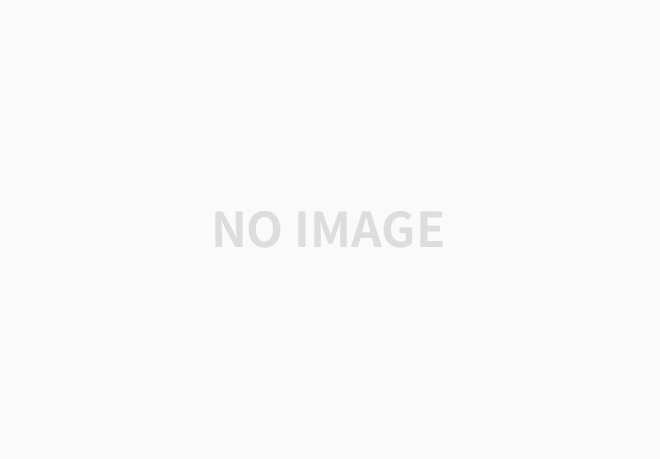
나의 접근법
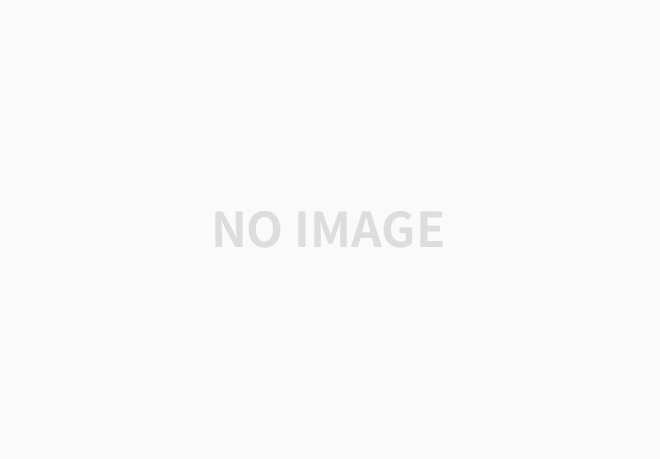
먼저 이해가 안되어서 문제를 그림으로 펼쳐서 주사위를 하나 하나 이동시키고 윗면(x표시)을 확인했습니다.
처음에 전개도가 나와서 시작부터 전개도 모양인줄 알고 따라했는데 도처히 예제처럼 답이 안나와서 헤맸는데 제가 문제를 제대로 읽지 않았습니다.
처음 시작은 주사위의 모든 면이 0 입니다!
그 후 각 이동 명령에서 주사위의 이동을 다음과 같이 표현하며 규칙이나 성질을 찾아봤습니다.
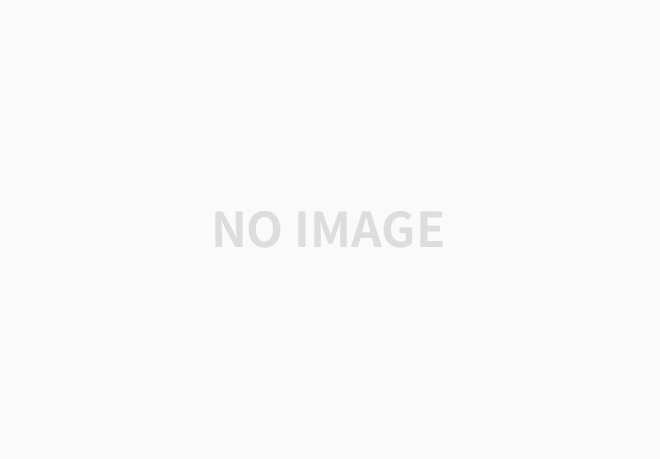
위와 같이 동쪽으로 이동할 경우 윗면이 동쪽으로 이동하므로 배열에서 4번으로 이동하게 됩니다.
동, 서, 남, 북 모두 규칙을 찾아 구현을 하니 쉽게 정답을 맞을 수 있었습니다.
제출 코드
def check(x, y):
if 0 <= x < N and 0 <= y < M:
return True
return False
N, M, x, y, K = map(int, input().split())
graph = []
for i in range(N):
graph.append(list(map(int, input().split())))
commands = list((map(int, input().split())))
dice = [0, 0, 0, 0, 0, 0, 0]
for cmd in commands:
if cmd == 1: # 동
nx, ny = x, y + 1
if check(nx, ny):
dice1, dice2, dice3, dice4, dice5, dice6 = dice[1], dice[2], dice[3], dice[4], dice[5], dice[6]
dice[1], dice[2], dice[3], dice[4], dice[5], dice[6] = dice4, dice2, dice1, dice6, dice5, dice3
if graph[nx][ny] == 0:
graph[nx][ny] = dice[6]
else:
dice[6] = graph[nx][ny]
graph[nx][ny] = 0
print(dice[1])
else:
continue
elif cmd == 2: # 서
nx, ny = x, y - 1
if check(nx, ny):
dice1, dice2, dice3, dice4, dice5, dice6 = dice[1], dice[2], dice[3], dice[4], dice[5], dice[6]
dice[1], dice[2], dice[3], dice[4], dice[5], dice[6] = dice3, dice2, dice6, dice1, dice5, dice4
if graph[nx][ny] == 0:
graph[nx][ny] = dice[6]
else:
dice[6] = graph[nx][ny]
graph[nx][ny] = 0
print(dice[1])
else:
continue
elif cmd == 3: # 북
nx, ny = x - 1, y
if check(nx, ny):
dice1, dice2, dice3, dice4, dice5, dice6 = dice[1], dice[2], dice[3], dice[4], dice[5], dice[6]
dice[1], dice[2], dice[3], dice[4], dice[5], dice[6] = dice5, dice1, dice3, dice4, dice6, dice2
if graph[nx][ny] == 0:
graph[nx][ny] = dice[6]
else:
dice[6] = graph[nx][ny]
graph[nx][ny] = 0
print(dice[1])
else:
continue
else: # 남
nx, ny = x + 1, y
if check(nx, ny):
dice1, dice2, dice3, dice4, dice5, dice6 = dice[1], dice[2], dice[3], dice[4], dice[5], dice[6]
dice[1], dice[2], dice[3], dice[4], dice[5], dice[6] = dice2, dice6, dice3, dice4, dice1, dice5
if graph[nx][ny] == 0:
graph[nx][ny] = dice[6]
else:
dice[6] = graph[nx][ny]
graph[nx][ny] = 0
print(dice[1])
else:
continue
x, y = nx, ny
후기
처음으로 검색이나 도움 없이 스스로 정답을 맞추었습니다. 사실 크게 어렵진 않아서 맞춘 것이 그렇게 기분이 좋지는 않았으나 계속 성장하는 느낌이 들었네요
다음에도 이렇게 큰 어려움 없이 해결했으면 좋겠습니다.
반응형
'ProblemSolving > 구현, 시뮬레이션, 완전탐색' 카테고리의 다른 글
백준 17779 게리맨더링2 (파이썬) (0) | 2022.04.13 |
---|---|
백준 17140 이차원 배열과 연산 (Python) (0) | 2022.04.11 |
백준 14500 테트로미노 - (Python) (0) | 2022.04.06 |
백준 12100 2048(Easy) - (Python) (0) | 2022.04.05 |
백준 5373 큐빙 -(Python) (0) | 2022.04.03 |